Building scalable applications from scratch involves several key steps and considerations to ensure that the application can handle increased load and user demands without significant performance degradation. Here’s a structured approach to building scalable applications:
1. Understand Requirements and Define Scalability Goals
- Understand the Use Case: Identify the core functionality, target audience, expected user base, and usage patterns.
- Set Scalability Goals: Define what “scalable” means for your application. Is it the ability to handle a certain number of concurrent users, process large amounts of data, or both?
2. Choose the Right Architecture
- Microservices Architecture: Consider breaking down the application into smaller, independent services that can be developed, deployed, and scaled independently.
- Serverless Architecture: Use serverless computing (e.g., AWS Lambda, Azure Functions) for applications that can benefit from event-driven execution and auto-scaling.
- Monolithic vs. Modular Monolith: If starting simple, a monolithic architecture can work, but ensure it’s modular enough to refactor into microservices if needed.
3. Select the Appropriate Tech Stack
- Languages and Frameworks: Choose languages and frameworks that are well-suited for scalability (e.g., Node.js, Python, Java, Go, etc.).
- Databases: Decide between SQL and NoSQL databases based on the data structure and scalability requirements. Consider options like PostgreSQL, MongoDB, or DynamoDB for horizontal scalability.
- Message Queues: Implement message queues (e.g., RabbitMQ, Kafka) for asynchronous processing and to decouple components.
4. Design for Scalability from the Ground Up
- Stateless Components: Design services to be stateless wherever possible, making it easier to scale horizontally by adding more instances.
- Data Partitioning: Use techniques like sharding or partitioning to distribute data across multiple databases or storage solutions.
- Caching Strategies: Implement caching mechanisms (e.g., Redis, Memcached) to reduce database load and improve response times.
- Load Balancing: Use load balancers to distribute traffic evenly across multiple servers or instances.
5. Implement Efficient Data Handling
- Optimize Queries: Use indexing, avoid N+1 query problems, and optimize database queries for better performance.
- Data Compression: Use data compression techniques to reduce the amount of data transferred and stored.
- Data Storage Solutions: Utilize cloud storage services (e.g., AWS S3, Google Cloud Storage) for scalable, durable, and cost-effective storage.
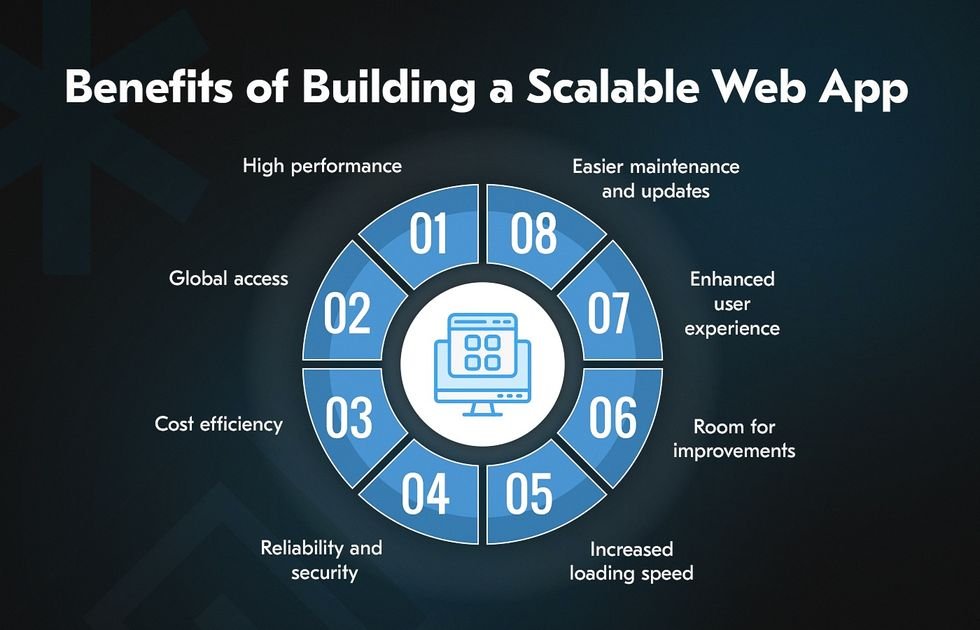
6. Focus on Performance Optimization
- Minimize Latency: Reduce network latency by deploying servers close to your user base (e.g., using Content Delivery Networks (CDNs)).
- Efficient Code: Write efficient code to minimize CPU and memory usage, which helps in handling more concurrent requests.
- Profiling and Monitoring: Continuously profile and monitor application performance to identify and resolve bottlenecks.
7. Ensure Robustness and Resilience
- Failover and Redundancy: Implement failover mechanisms and redundancy to ensure the application remains available during failures.
- Circuit Breaker Pattern: Use circuit breaker patterns to handle failures gracefully and prevent cascading failures across the system.
- Auto-Scaling: Utilize auto-scaling features provided by cloud providers to automatically adjust resources based on demand.
8. Automate Deployment and Testing
- CI/CD Pipelines: Implement Continuous Integration and Continuous Deployment (CI/CD) pipelines to automate testing, deployment, and scaling.
- Infrastructure as Code (IaC): Use tools like Terraform or AWS CloudFormation to manage infrastructure as code, making it easier to replicate and scale environments.
- Automated Testing: Include automated unit, integration, and load testing to ensure the application is robust and scalable.
9. Monitor and Manage Resources
- Logging and Monitoring: Use centralized logging (e.g., ELK Stack) and monitoring solutions (e.g., Prometheus, Grafana) to track performance metrics and detect anomalies.
- Alerting: Set up alerts for critical metrics to proactively manage issues before they affect end users.
- Capacity Planning: Regularly review capacity and plan for scaling up or down based on usage trends.
10. Plan for Continuous Improvement
- Feedback Loops: Gather feedback from users and stakeholders to continuously improve the application’s performance and scalability.
- Stay Updated: Keep up with new technologies, tools, and best practices to enhance the scalability and performance of your application.
Conclusion
Building a scalable application requires careful planning, choosing the right technologies, designing for scalability from the start, and continuously monitoring and optimizing performance. By following these steps, you can build applications that handle growth effectively and provide a seamless user experience even under high load.

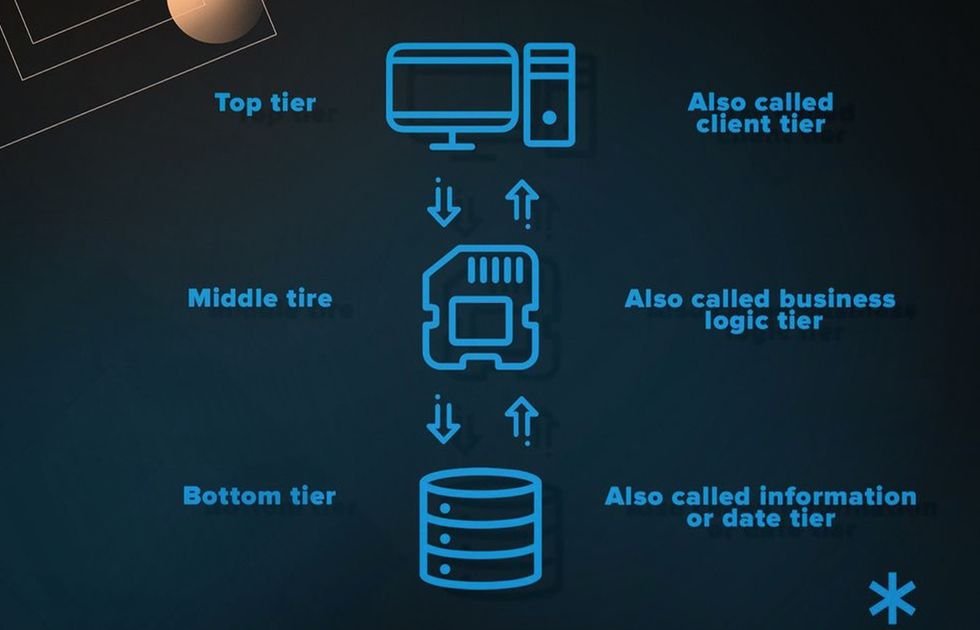
Good